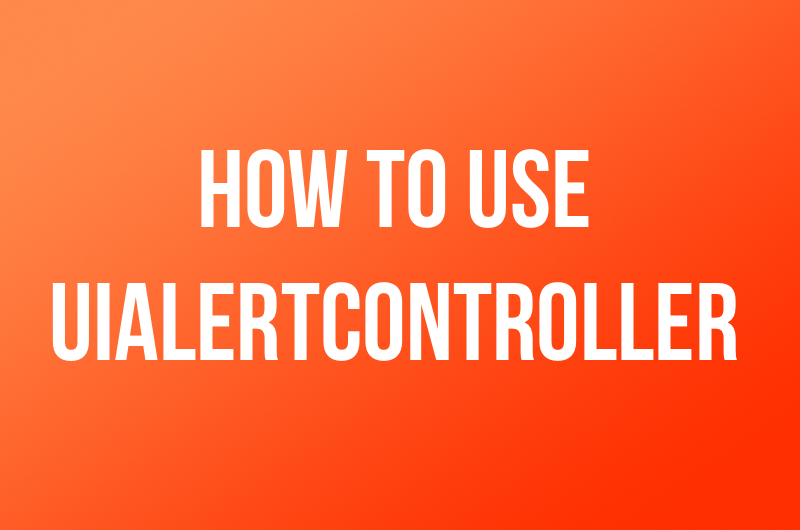
How To Use UIAlertController in iOS
If your reading this, then you stumbled on to my next post since writing my introductory post on New Years Day. If you have not read that yet, I strongly suggest you do that to learn more about why I’m blogging. Anyway, today’s post is all about some recent findings I discovered during the development process of the ParkLane iOS app. My use case is pretty simple and common for the platform. I have a login and sign up ViewControllers inside my app where the user can fill out some fields to create an account in our database or login into a existing one. However, I needed some kind of alert to pop up on the screen to tell a user if one of the following errors occurred:
- Wrong Email
- Wrong Password
- Wrong Email and Password
As you can tell this presents a problem. I know Apple has something to solve this called a UIAlertView
. Its pretty simple to use, it looks like this:
let button2Alert: UIAlertView = UIAlertView(title: "Title", message: "message",
delegate: self, cancelButtonTitle: "Ok", otherButtonTitles: nil)
// show alert on screen
button2Alert.show()
I wasn’t worried to use this code because I’ve used it before, However Xcode presented me with this warning:
UIAlertView is deprecated. Use UIAlertController with a preferredStyle of UIAlertControllerStyleAlert instead
This left me puzzled, after doing some research this change occurred around the release of iOS 8 in 2014. After thinking about it some more, It must have been a change that I simply missed, and I take the blame for it. In a request to redeem myself I took out the old UIAlert code and replaced it with the newer UIAlertController
.
After trying this new API, I have to say I like this better than the old UIAlertView. The newer framework seems like more work, but your getting more control over your alerts. Here is a quick code example to show you the difference.
let alert = UIAlertController(title: "Alert", message: "Message", preferredStyle: UIAlertControllerStyle.Alert)
alert.addAction(UIAlertAction(title: "Click", style: UIAlertActionStyle.Default, handler: nil))
self.presentViewController(alert, animated: true, completion: nil)
If your familiar with how iOS views work the this seems pretty straight forward. I bet developers that don’t understand iOS or Swift can figure out what this code does. I also enjoy that the addAction
method has a completion handler in order to execute some custom code when you tap a button on the alert.
Before I go, I realize that this first iOS post was pretty simple but do not worry. I have plenty of content coming up on more advance topics. So keep following my blog and be sure to follow me on Twitter to be alerted when new content is posted.